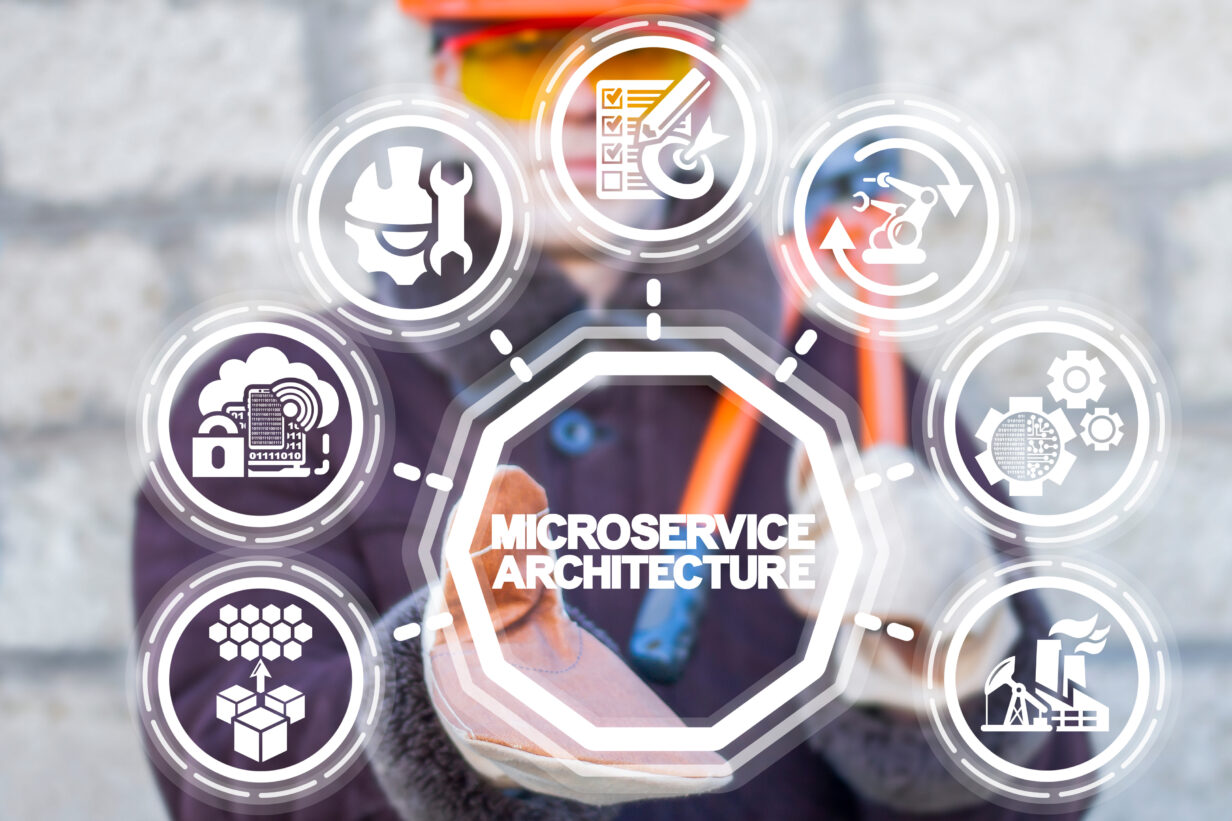
As a software engineer working with .NET, integrating cloud-native technologies into your local development workflow can be challenging. However, with Dapr (Distributed Application Runtime), you can simplify the process and run a “cloud on your box.” In this detailed blog post, we’ll delve deeper into how you can leverage Dapr to streamline .NET development, covering setup, core components, and practical examples.
What is Dapr?
Dapr is an open-source, portable, and event-driven runtime designed to make it easier to build microservices and cloud-native applications. It provides a set of building blocks that abstract common tasks like service invocation, state management, pub/sub messaging, and more, allowing developers to focus on business logic rather than infrastructure complexities.
How Dapr Abstracts Cloud-Native Technology to Deliver the Best Developer Experience
Dapr’s core philosophy is to provide a set of building blocks that encapsulate common microservice patterns, thereby abstracting the underlying cloud-native infrastructure. This abstraction allows developers to focus on writing business logic rather than dealing with the intricacies of distributed systems. Here’s a closer look at how Dapr achieves this and enhances the developer experience:
1. Service Invocation Abstraction
Service-to-service communication in a microservices architecture often involves dealing with complex networking issues, retries, timeouts, and circuit-breaking policies. Dapr abstracts these complexities through its service invocation API, enabling secure and reliable communication between services using simple HTTP or gRPC calls. Developers no longer need to implement these low-level details themselves, significantly reducing the boilerplate code and potential for errors.
2. Simplified State Management
Managing state in a distributed system is notoriously difficult, with challenges such as consistency, latency, and scalability. Dapr provides a consistent state management API that works with a variety of state stores, including Redis, Azure Cosmos DB, and AWS DynamoDB. This abstraction allows developers to store and retrieve state without worrying about the specifics of the underlying storage mechanism. As a result, building stateful applications becomes as straightforward as using a local key-value store.
3. Decoupled Communication with Pub/Sub
Dapr’s publish/subscribe component abstracts the details of message brokers, allowing services to communicate asynchronously and decouple from one another. By supporting multiple message brokers like Kafka, RabbitMQ, and Azure Service Bus, Dapr ensures that developers can easily switch between different pub/sub implementations without changing their application code. This flexibility enhances scalability and robustness, enabling developers to focus on the logic of event-driven systems.
4. Streamlined Resource Bindings
Interacting with external systems such as databases, queues, and file systems often requires extensive configuration and integration code. Dapr’s binding component abstracts these integrations by providing a common interface for input and output bindings. Developers can easily connect their applications to external resources without writing custom connectors, thereby speeding up development and reducing the potential for bugs.
5. Built-in Observability
Observability is crucial for maintaining and debugging distributed systems, yet implementing comprehensive logging, metrics, and tracing can be complex and time-consuming. Dapr includes built-in observability features that abstract these concerns, providing automatic instrumentation for tracing, logging, and monitoring. This enables developers to gain deep insights into their application’s behavior and performance with minimal configuration, ensuring high availability and quick issue resolution.
6. Portability Across Environments
One of Dapr’s most significant advantages is its portability. Whether running on a local development machine, on-premises, or in the cloud, Dapr ensures a consistent developer experience. Developers can run their entire microservice architecture locally using Dapr, simulating a cloud environment (“cloud on your box”) and allowing for comprehensive development and testing before deployment. This consistency across environments eliminates the “it works on my machine” problem and streamlines the CI/CD pipeline.
Setting Up Dapr for .NET Development
To get started with Dapr in a .NET environment, follow these steps:
1. Install Prerequisites
Before installing Dapr, ensure you have the following installed on your machine:
2. Initialize Dapr
Once you have the prerequisites installed, initialize Dapr using the CLI:
dapr init
This command sets up the necessary Dapr components, including a Redis state store, a placement service for actors, and a Zipkin instance for tracing.
3. Create a .NET Application
Let’s create a simple .NET web API project to demonstrate how to integrate Dapr:
dotnet new webapi -n DaprDemo
cd DaprDemo
4. Add Dapr SDK to Your Project
To interact with Dapr components, add the Dapr .NET SDK to your project:
dotnet add package Dapr.Client
Utilizing Dapr Building Blocks in .NET
Dapr provides several building blocks that simplify common cloud-native patterns. Let’s explore how to use these building blocks in a .NET application.
Service Invocation
Dapr simplifies service-to-service invocation with HTTP and gRPC. Here’s how you can call another service using Dapr in a .NET controller:
- Create a Controller: Create a new controller called
InvokeController
:
using Dapr.Client;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace CK.DaprDemo
.Controllers
{
[ApiController]
[Route("[controller]")]
public class InvokeTestController : ControllerBase
{
private readonly DaprClient _daprClient;
public InvokeTestController
(DaprClient daprClient)
{
_daprClient = daprClient;
}
[HttpGet("{method}")]
public async Task<IActionResult> InvokeMethodAsync(string method)
{
var response = await _daprClient.InvokeMethodAsync<string>(HttpMethod.Get, "myapp", method);
return Ok(response);
}
}
}
- Run the Application with Dapr: Use the following command to run your application with Dapr:
dapr run --app-id daprdemo --app-port 5000 -- dotnet run
Now you can call another service’s endpoint using the Dapr client.
State Management
Dapr’s state management API allows you to store and retrieve state seamlessly. Here’s how to use it in a .NET application:
- Create a State Management Controller: Add a new controller called
StateController
:
using Dapr.Client;
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
using System.Threading.Tasks;
namespace CK.DaprDemo
.Controllers
{
[ApiController]
[Route("[controller]")]
public class StateController : ControllerBase
{
private readonly DaprClient _daprClient;
public StateController(DaprClient daprClient)
{
_daprClient = daprClient;
}
[HttpPost]
public async Task<IActionResult> SaveStateAsync([FromBody] KeyValuePair<string, string> state)
{
await _daprClient.SaveStateAsync("statestore", state.Key, state.Value);
return Ok();
}
[HttpGet("{key}")]
public async Task<IActionResult> GetStateAsync(string key)
{
var state = await _daprClient.GetStateAsync<string>("statestore", key);
return Ok(state);
}
}
}
- Run the Application with Dapr: Use the following command to run your application with Dapr:
dapr run --app-id daprdemo --app-port 5000 -- dotnet run
You can now save and retrieve state using Dapr’s state management API.
Publish/Subscribe
Dapr’s pub/sub component enables decoupled communication between services. Here’s how to implement it in a .NET application:
- Create a Pub/Sub Controller: Add a new controller called
PubSubController
:
using Dapr.Client;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace CK.DaprDemo
.Controllers
{
[ApiController]
[Route("[controller]")]
public class PubSubController : ControllerBase
{
private readonly DaprClient _daprClient;
public PubSubController(DaprClient daprClient)
{
_daprClient = daprClient;
}
[HttpPost]
public async Task<IActionResult> PublishMessageAsync([FromBody] string message)
{
await _daprClient.PublishEventAsync("pubsub", "mytopic", message);
return Ok();
}
[Topic("pubsub", "mytopic")]
[HttpPost("subscribe")]
public IActionResult SubscribeMessage([FromBody] string message)
{
return Ok($"Received message: {message}");
}
}
}
- Configure Pub/Sub Component: Create a
components
directory in your project root and add a file namedpubsub.yaml
:
apiVersion: dapr.io/v1alpha1
kind: Component
metadata:
name: pubsub
namespace: default
spec:
type: pubsub.redis
version: v1
metadata:
- name: redisHost
value: "localhost:6379"
- Run the Application with Dapr: Use the following command to run your application with Dapr:
dapr run --app-id daprdemo --app-port 5000 --components-path ./components -- dotnet run
You can now publish and subscribe to messages using Dapr’s pub/sub API.
Enhanced Observability with Dapr
Dapr’s observability features, including metrics, tracing, and logging, help you monitor and troubleshoot your applications. Here’s how to enable tracing in a .NET application:
- Configure Tracing: Create a
tracing.yaml
file in thecomponents
directory:
apiVersion: dapr.io/v1alpha1
kind: Configuration
metadata:
name: tracing
spec:
tracing:
samplingRate: "1"
- Apply the Configuration: Use the following command to apply the configuration:
dapr apply -f components/tracing.yaml
- Run the Application with Dapr: Use the following command to run your application with Dapr:
dapr run --app-id daprdemo --app-port 5000 --components-path ./components --config ./components/tracing.yaml -- dotnet run
With tracing enabled, you can monitor your application’s performance and troubleshoot issues using Dapr’s built-in observability tools.
Conclusion
Dapr is a game-changer for .NET developers looking to build cloud-native applications locally. By abstracting the complexities of service invocation, state management, pub/sub messaging, and observability, Dapr allows you to focus on writing business logic and provides a consistent developer experience across different environments. Embrace the power of Dapr and transform your local development workflow into a seamless, cloud-like experience. With Dapr, you truly have the cloud at your fingertips, right on your local machine.
No Comments